A palindrome is a word, phrase, or sequence that reads the same backward as forward, ignoring spaces, punctuation, and capitalization. Examples include “madam,” “racecar”.
In this tutorial, you’ll learn how to build a Python program to detect palindromes. This lab is perfect for beginners looking to practice string manipulation, conditionals, and functions in Python.
Step 1: Plan the Program
Define what our program will do:
- Define a function
- Removing spaces and punctuation in words like “race car”.
- Converting all characters to lowercase.
- Joint the split components to make one word.
- Reverse the string to compare it to the original.
- Check if the normalized string reads the same backward as forward
- Display whether the word is a palindrome.
Step 2: Set Up the Environment
Make sure you have Python installed. You can use any code editor or an IDE like VS Code, PyCharm, or Jupyter Notebook.
Step 3: Write the Code
Below is the Python code for the palindrome detector:
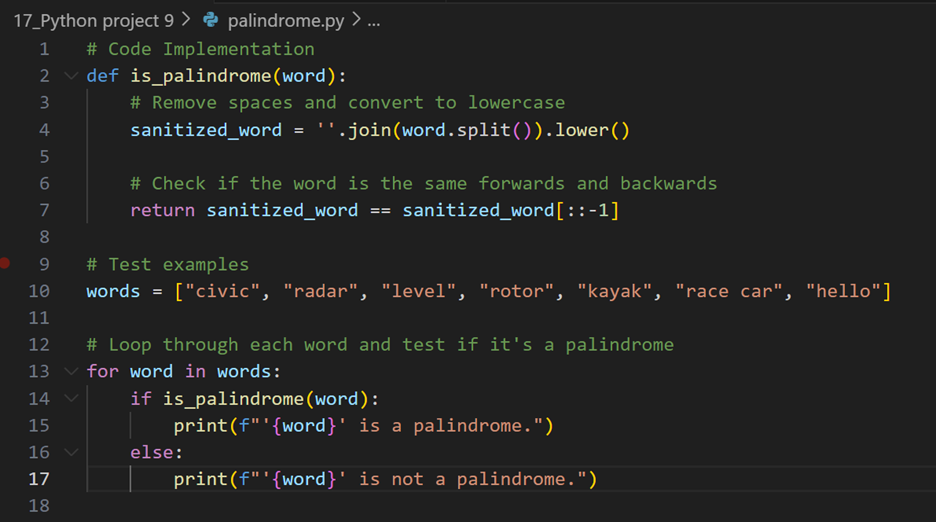
Step 4: Testing the Program
Run the program and try the following inputs:
- Single words: civic, radar, level, rotor, kayak.
- Phrases with spaces and punctuation: race car.
- Random word: hello
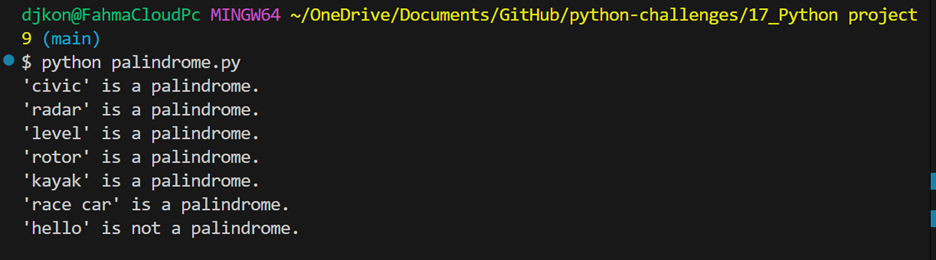
This Python program is a great way to practice string manipulation and conditional logic. Whether you’re a beginner or brushing up on Python skills, building this palindrome detector is both fun and educational.
Now it’s your turn. Try implementing the code, testing with your own examples, and even adding enhancements to make it more robust.
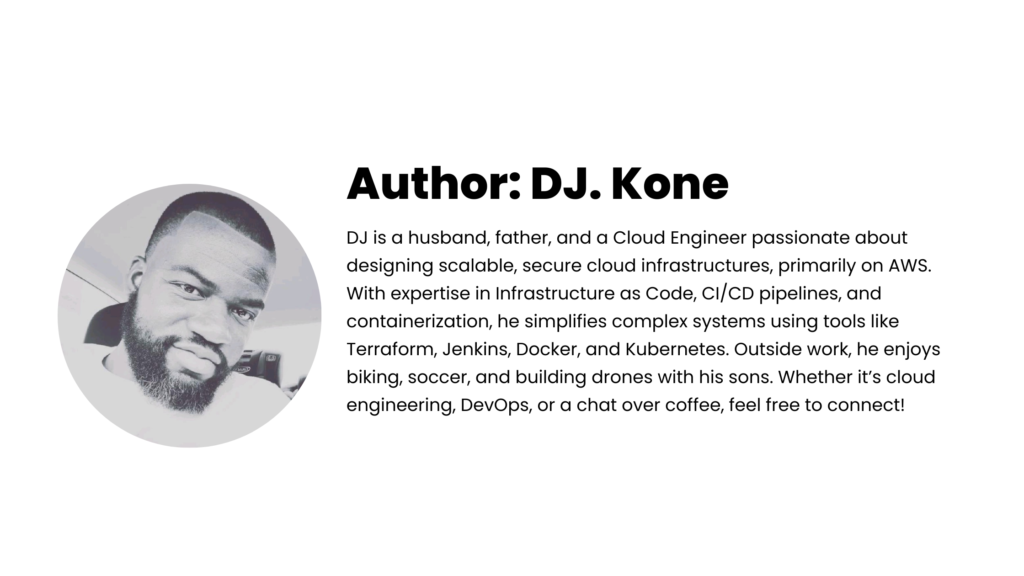
Leave a Reply