In this tutorial, I will show you how Boto3 can be used to directly interact with AWS resources from Python scripts.
If you’re diving into AWS with Python, Boto3 is the go-to library. It’s the official SDK that makes it easy to interact with AWS resources directly from your Python scripts. In this guide, I’ll walk through how to use Boto3 to handle various tasks with AWS Identity and Access Management (IAM).
With IAM, you can:
- Create new users
- Manage permissions
- Set up policies
- And so much more.
Prerequisite
Before we dive into the code, make sure you’ve got these set up:
- Python 3: Install it if you don’t already have it.
- Boto3: You can install it with pip: pip install boto3.
- AWS Credentials: You’ll need these to access your AWS account. See below:

How to create a user
When you set up a new AWS account, it’s a best practice to create a dedicated IAM user instead of using your root account for day-to-day tasks.
- create a python file createuser.py with the code below in the file
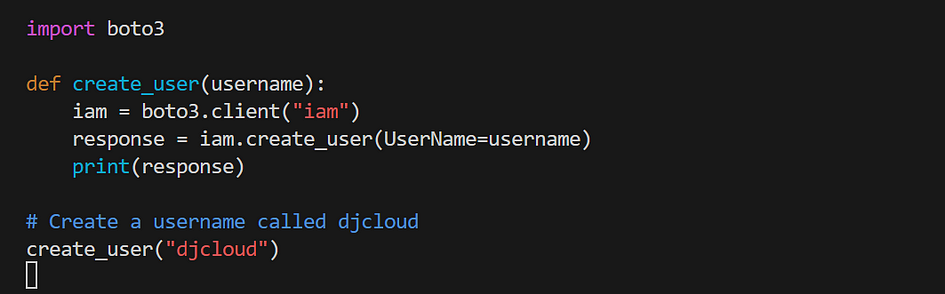
- Run python createuser.py
- You should see this below in your terminal.

- Go to your AWS console, you should see the user has been created.
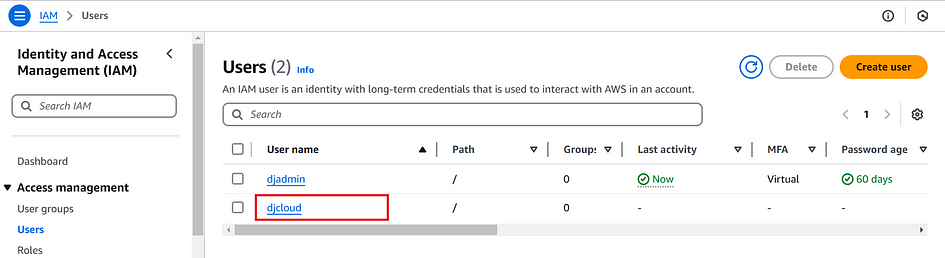
How to List All IAM Users
- create a python file with the following code
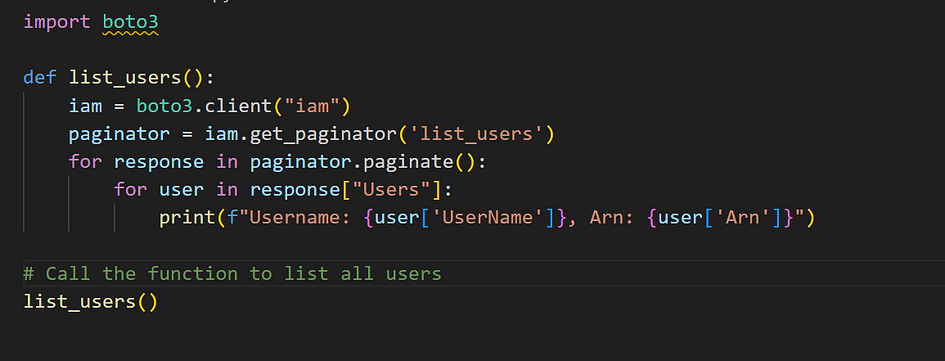
- Run it. Here is the result.

How to Update a User
Need to rename an IAM user? AWS lets you update a user’s details with Boto3’s update_user
function. This is especially useful when renaming users to align with new naming conventions or correcting a mistake.
- create a python file with this code
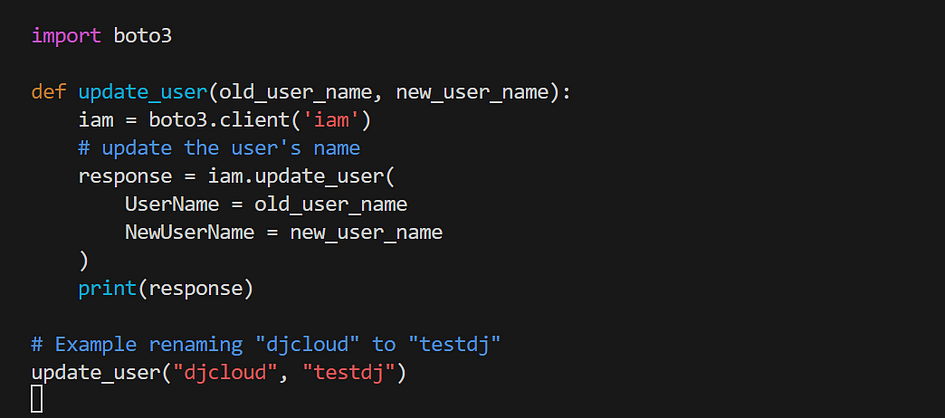
- Here is the result in your terminal.

- You should see the change in your aws console.
How to Create an IAM Policy
An IAM policy defines the actions a user or group can perform and the resources those actions apply to. With Boto3, you can create custom policies tailored to your specific access control needs. Let’s walk through creating a policy that grants access to specific actions in DynamoDB.
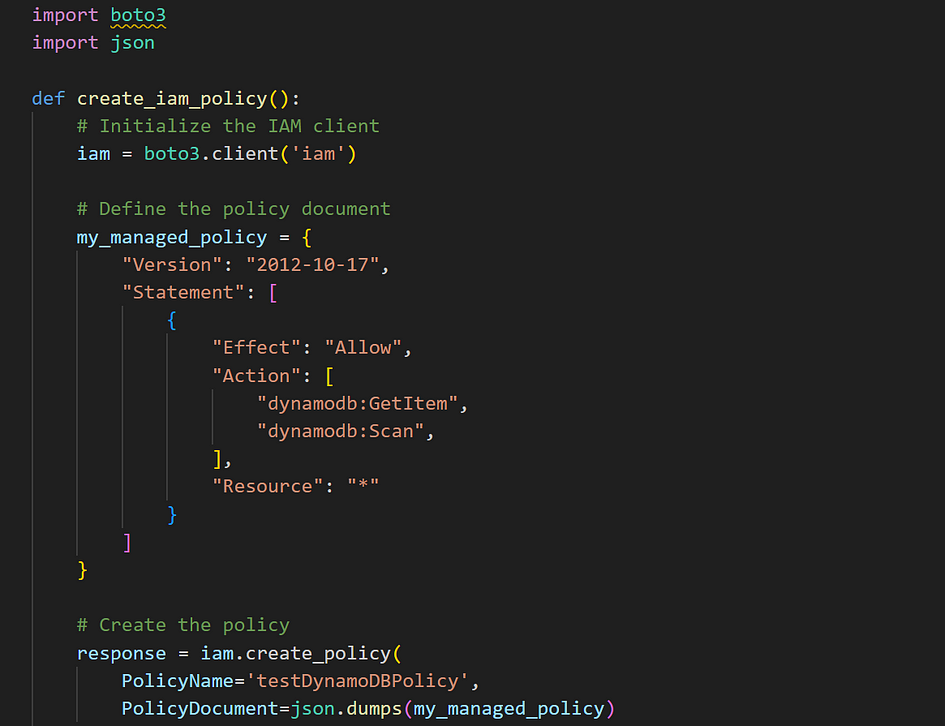
- Go to the IAM console. Click on poloicies and see new policy.
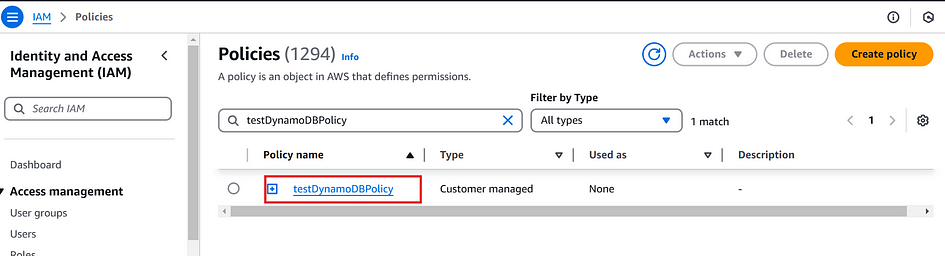
How to List All IAM Policies
To efficiently manage permissions in your AWS account, it’s essential to know which IAM policies exist. Using Boto3, we can retrieve a list of IAM policies, filtering for those created within your account.
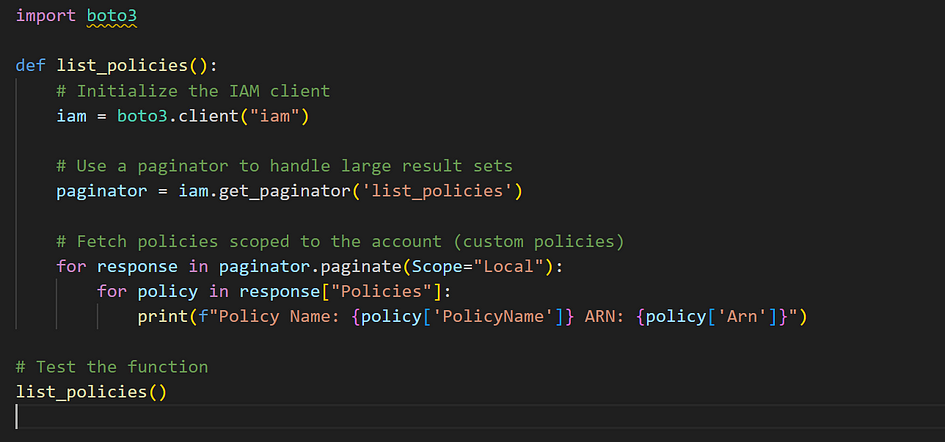
How to Attach a Policy to a User
Attaching IAM policies to individual users is essential for granting specific permissions. Below is a step-by-step guide using Boto3 to attach a policy to a user.
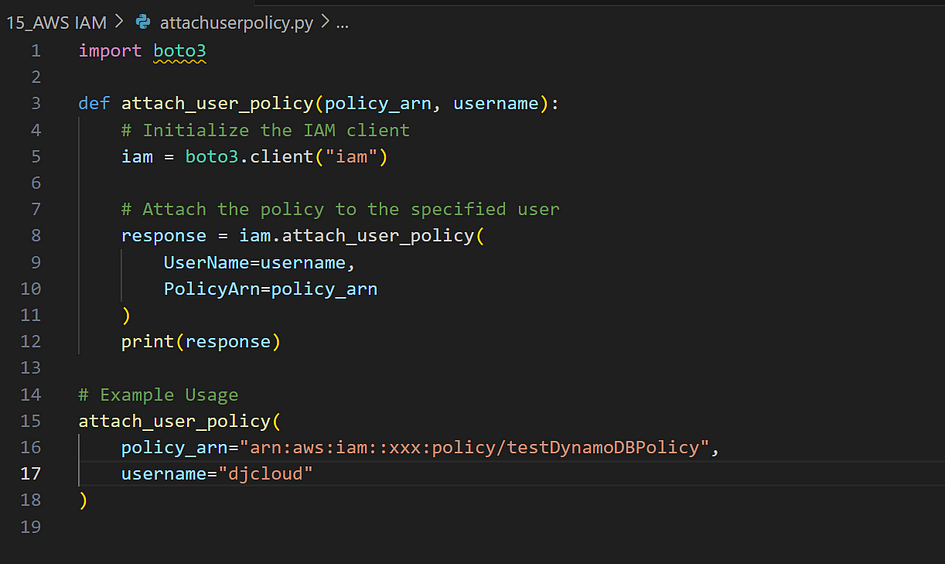
How to Create a Group
When managing permissions in AWS, creating groups helps streamline the process. Instead of attaching policies to individual users, you can attach policies to a group. All users added to the group inherit the permissions of the group’s policies.
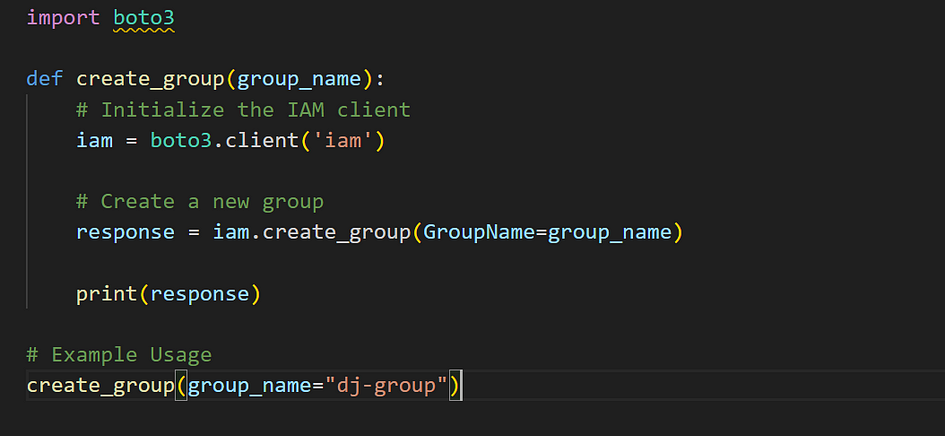
How to Add a User to a Group
In AWS Identity and Access Management (IAM), users can be added to groups to inherit the permissions attached to the group. This is an efficient way to manage user permissions across your AWS account.
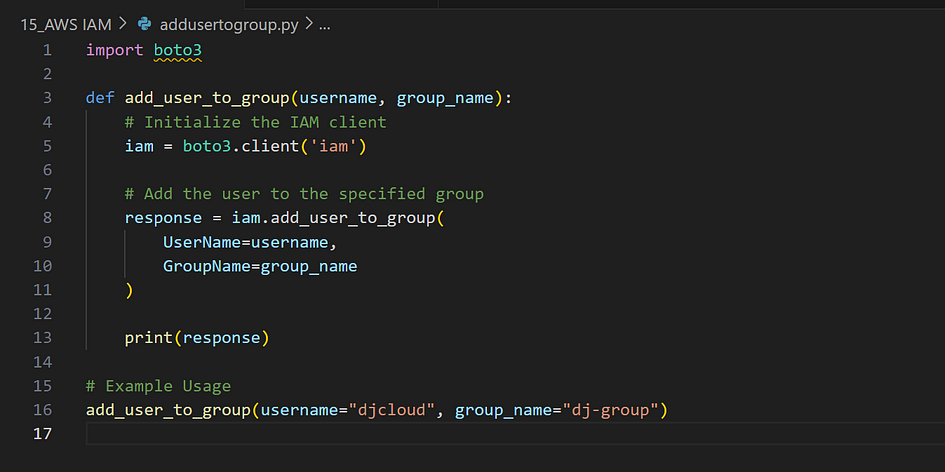
How to Attach an IAM Policy to a Group
Attaching a policy to a group allows all users in that group to inherit the permissions defined in the policy. This streamlines permission management for multiple users.
Find the python code here: https://github.com/djcloudking/aws-skills-challenges/blob/main/15_AWS%20IAM/attachpolicytogroup.py
How to Create an IAM Role
An IAM role is a secure way to grant permissions to AWS services or users. The creation of a role requires defining a trust relationship policy to specify which entities (such as AWS services) can assume the role.
Find the python code here: https://github.com/djcloudking/aws-skills-challenges/blob/main/15_AWS%20IAM/iamrole.py
How to Attach an IAM Policy to a Role
An IAM policy defines the permissions for an IAM role. You can attach policies to a role using the attach_role_policy
method provided by the AWS SDK (boto3
).
Find the python code here: https://github.com/djcloudking/aws-skills-challenges/blob/main/15_AWS%20IAM/attachiampolicytorole.py
— — — — —
Thank you for reading and/or following along! Leave us a clap or a comment, Share & Follow. Please stay tuned for all my upcoming projects.
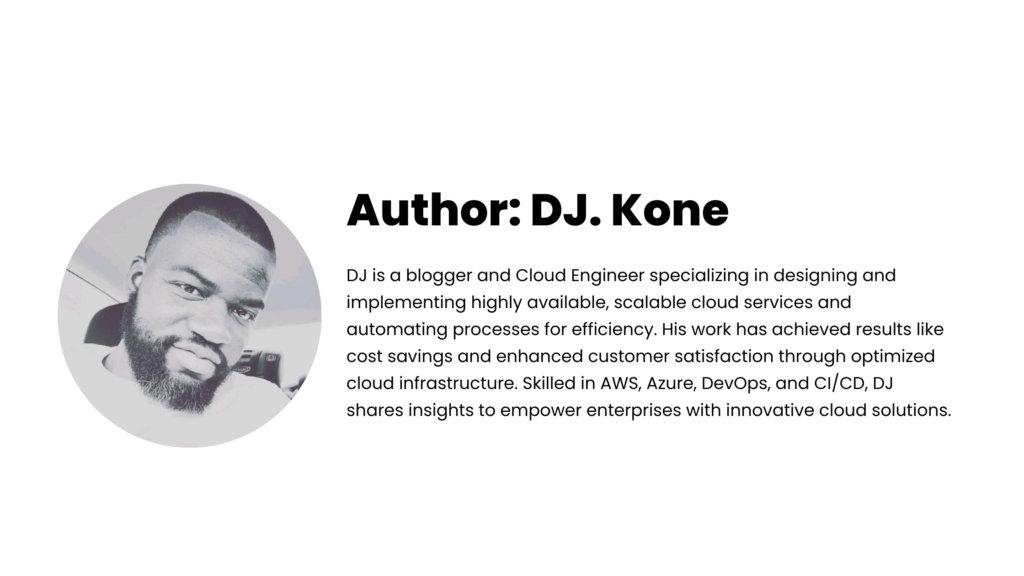
Leave a Reply